Introduction
This document describes a simple approach of implementing AJAX functionality in ASP.NET web applications. Pros and cons of using AJAX are also discussed. The document contains a working JavaScript and C#.NET code demonstrating the suggested solution.
Why AJAX
Most of you already know that AJAX stands for Asynchronous JavaScript and XML. This technology was introduced first by Microsoft (from my best knowledge) back in 1999, and had been known as “DHTML / JavaScript web application with remote calls”. The whole idea of the technology was to allow an internet browser to make an asynchronous HTTP call to remote pages/services, and update a current web page with the received results without refreshing the whole page. By creators’ opinion, this should have improved customers’ experience, making HTTP pages look and feel very similar to Windows applications.
Because the core implementation of this technology was based on internet browser functionality, the usability was very limited at that time. But several years later, the technology has been reborn with new browsers support and massive implementation by such giants as Google, Amazon.com, eBay, etc.
Today, it’s known as AJAX, and considered as a natural part of any dynamic web page with advanced user experience.
Solution Description
The suggested approach provides a very simple, yet effective implementation of the AJAX functionality. It’s very easy to maintain and change, does not require any special skills from developers, and, from our best knowledge, is cross-browser compatible.
Basically, a regular AJAX-like implementation includes two main components: a client HTML page with JavaScript code making an AJAX call and receiving a response, and a remote page that can accept a request and respond with the required information. The JavaScript code on the client page is responsible for instantiating an XmlHttp
object, then providing this object with a callback method which will be responsible for processing the received information, and finally, sending a request to the remote page via the XmlHttp
object. All this is done by the JavaScript code.
Our approach is intended for use in ASP.NET applications, and considers the following possible scenarios:
- AJAX calls may be performed on different ASP.NET pages of the web application to different remote pages;
- A remote page URL may contain dynamically calculated parameters, and it may be more convenient to build a URL string in the code-behind of the ASP.NET page;
- A remote page may respond with a complex data requiring parsing before updating an HTML page, that once again may be done in the code-behind of the ASP.NET page;
- A remote page may be either an external third party page, or the web application’s own page or service.
All these considerations are illustrated by the diagram below:
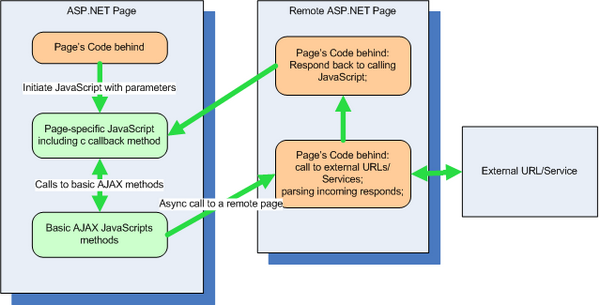
Implementation
I divided all the JavaScript methods into two parts: calling page specific JavaScript methods, and AJAX JavaScript methods common for all the calling pages. Specific methods include a callback method as well, as it is responsible for updating the page content. Common AJAX methods are responsible for instantiating an XmlHttp
object and sending an asynchronous request to the remote page.
Getting an XmlHttp
object differs depending on the type of the browser. I distinguish two basic types: a Microsoft browser which is one of the IE family, and a Mozilla browser which is one of Mozilla Firefox, Netscape, or Safari. I tested the code with the Opera browser too, but I would not guarantee that it will always be working well.
Collapsefunction GetXmlHttpObject(handler)
{
var objXmlHttp = null;
if (!window.XMLHttpRequest)
{
objXmlHttp = GetMSXmlHttp();
if (objXmlHttp != null)
{
objXmlHttp.onreadystatechange = handler;
}
}
else
{
objXmlHttp = new XMLHttpRequest();
if (objXmlHttp != null)
{
objXmlHttp.onload = handler;
objXmlHttp.onerror = handler;
}
}
return objXmlHttp;
}
function GetMSXmlHttp()
{
var xmlHttp = null;
var clsids = ["Msxml2.XMLHTTP.6.0","Msxml2.XMLHTTP.5.0",
"Msxml2.XMLHTTP.4.0","Msxml2.XMLHTTP.3.0",
"Msxml2.XMLHTTP.2.6","Microsoft.XMLHTTP.1.0",
"Microsoft.XMLHTTP.1","Microsoft.XMLHTTP"];
for(var i=0; i<clsids.length && xmlHttp == null; i++) {
xmlHttp = CreateXmlHttp(clsids[i]);
}
return xmlHttp;
}
function CreateXmlHttp(clsid) {
var xmlHttp = null;
try {
xmlHttp = new ActiveXObject(clsid);
lastclsid = clsid;
return xmlHttp;
}
catch(e) {}
}
According to Umut Alev, the code for the GetMSXmlHttp
method can be simplified considering that we do not have to refer MSXML5 as it has been designed only for Office applications. Correspondingly, the simplified revision of the GetMSXmlHttp
method may look as follows:
function GetMSXmlHttp() {
var xmlHttp = null;
var clsids = ["Msxml2.XMLHTTP.6.0",
"Msxml2.XMLHTTP.4.0",
"Msxml2.XMLHTTP.3.0"];
for(var i=0; i<clsids.length && xmlHttp == null; i++) {
xmlHttp = CreateXmlHttp(clsids[i]);
}
return xmlHttp;
}
As you see, GetXmlHttpObject
methods accept a handler parameter which is a name of the callback method that should be defined in the page-specific code. Now that we already have an XmlHttp
object, we can send an asynchronous request.
function SendXmlHttpRequest(xmlhttp, url) {
xmlhttp.open('GET', url, true);
xmlhttp.send(null);
}
I use a GET HTTP method to a given URL, but this can be easily changed by changing the JS code above.
Now we have all the methods we need to perform a call to the remote page. In order to do this, we need to pass the callback method name to the GetXmlHttpObject
method and then pass the URL string to the SendXmlHttpRequest
method.
Collapsevar xmlHttp;
function ExecuteCall(url)
{
try
{
xmlHttp = GetXmlHttpObject(CallbackMethod);
SendXmlHttpRequest(xmlHttp, url);
}
catch(e){}
}
function CallbackMethod()
{
try
{
if (xmlHttp.readyState == 4 ||
xmlHttp.readyState == 'complete')
{
var response = xmlHttp.responseText;
if (response.length > 0)
{
document.getElementById("elementId").innerHTML
= response;
}
}
}
catch(e){}
}
The CallbackMethod
is responsible for updating the page content. In our example, it simply updates the inner HTML of the given HTTP element. But in real life, it can be much more complex.
The last question regarding the calling page implementation is how we call the ExecuteCall
JS method. Well, it depends on what the page is doing. In some cases, the ExecuteCall
method can be called when the JS event is fired. But if that is not the case, we can register the method as a startup script for the page using the corresponding C# code in the page's code-behind.
Page.RegisterStartupScript("ajaxMethod",
String.Format("<script>ExecuteCall('{0}');</script>", url));
We can add this line of code either in the Page_Prerender
or Page_Load
method of the ASP.NET code-behind file.
Let’s find out what a remote page could look like. If this is an ASP.NET page (what we assume), we are interested in the code-behind only. We can easily remove all the code from the .aspx file: it won’t affect the behavior of the page in any way.
For example, we take a public web service that converts temperature values in Celsius to Fahrenheit and vice versa. The service is available here. If you add this URL as a web reference to your project, Visual Studio will generate a proxy class with the name com.developerdays.ITempConverterservice
in your current namespace. Our remote ASP.NET page, let’s name it getTemp.aspx, will accept a query string parameter with the name “temp
” which should contain an integer value of a temperature in Celsius to convert. So the target URL to the remote page will look like this: http://localhost/getTemp.aspx?temp=25. And the code-behind for this page is shown below:
private void Page_Load(object sender, EventArgs e)
{
Response.Clear();
string temp = Request.QueryString["temp"];
if (temp != null)
{
try
{
int tempC = int.Parse(temp);
string tempF = getTempF(tempC);
Response.Write(tempF);
}
catch
{
}
}
Response.End();
}
private string getTempF(int tempC)
{
com.developerdays.ITempConverterservice
svc = new ITempConverterservice();
int tempF = svc.CtoF(tempC);
return tempF.ToString();
}
According to our convention, we can now build a URL string for the remote page that we will pass to the RegisterStartupScript
method in the example above, like this:
int tempC = 25;
string url = String.Format("http://localhost/" +
"getTemp.aspx?temp={0}", tempC);
Using the approach with an intermediate ASP.NET page, calling in its turn a remote service, allows simplifying response processing, especially if it requires parsing. In simple cases when the response contains just text, we can pass the remote service URL directly to the JS ExecuteCall
method.
This article is aimed to illustrate the simplicity of using the AJAX technology in any ASP.NET application. While AJAX has some drawbacks, it also provides some advantages from the user experience perspective. It’s completely up to the developer whether to use the AJAX technology or not, but I have just demonstrated that in simple cases, it does not take a long time or require any special skills.
Credits
I would like to thank my colleagues Oleg Gorchkov and Chris Page who helped me out with testing and optimizing the approach described in the article.